Breaking Access Barriers: My Journey into App Security
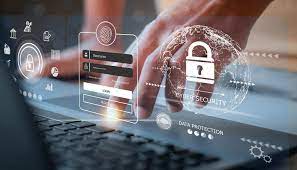
You know that satisfying moment when you’re deep in the code... squashing bugs, pushing out features, and feeling like you’ve got it all under control? That was me. Fully immersed in dev life, convinced I had everything sorted.
Then, out of the blue, I came across something that completely changed how I think about building applications: broken access control.
It’s one of the most common (and most dangerous) security flaws out there. And once you see how easily it can be exploited, you can’t unsee it.
Let me walk you through what I learned... how broken access control works, what it looks like in the real world, and most importantly, how to protect your applications against it.
What Is Broken Access Control?
Put simply, access control is about defining who can do what in your app. Sounds straightforward enough, right? But if you don’t lock things down properly, you risk:
- Data leaks
- Unauthorised access
- Even full system compromise
Here are some of the usual culprits when access control isn’t handled correctly:
- Least Privilege Violation – Users having more access than necessary
- URL Manipulation – Changing URL parameters to sneak into areas they shouldn’t
- Unauthorised Account Access – Viewing or modifying another user’s data
- Weak API Security – APIs not verifying permissions or roles properly
- Token Tampering – Modifying JWTs or session tokens to gain higher privileges
- CORS Misconfiguration – Letting dodgy origins talk to your APIs
- Forced Browsing – Accessing admin pages without even logging in
I witnessed these flaws in action during a hands-on security workshop. Watching someone bypass login screens just by tweaking a URL or injecting a bit of SQL? It was eye-opening.
Real-World Examples That Gave Me Chills
SQL Injection to Access Other Accounts
Imagine you’ve got a web app that pulls up user info using a URL like:
https://example.com/app/accountInfo?acct=123456
An attacker changes it to:
https://example.com/app/accountInfo?acct=999999 OR 1=1
If your backend isn’t secure, the resulting SQL might look like:
SELECT * FROM accounts WHERE account_number = '999999' OR 1=1;
And just like that, the attacker can see everyone’s account data.
Accessing Admin Features Without Being an Admin
Consider these API endpoints:
/readonly_getappInfo
/admin_getappInfo
If someone who isn’t an admin can simply type in the admin URL and get access, that’s a serious red flag. No fancy hacking tools needed... just a bit of curiosity and broken access control.
How to Fix It
Here’s the access control checklist I now follow:
- Deny all access by default... explicitly allow only what’s needed
- Implement role-based access control (RBAC)
- Log failed access attempts and alert on suspicious behaviour
- Apply rate limiting to deter brute-force attempts
- Secure your tokens... make them short-lived and revoke on logout
Implementing Role-Based Access in Flask
Here’s a simple Flask example showing how to enforce access control in an API:
from flask import Flask, request, jsonify
app = Flask(__name__)
users = {
'alice': {'role': 'user', 'id': 1},
'bob': {'role': 'admin', 'id': 2},
}
@app.route('/user/<username>', methods=['GET'])
def get_user_data(username):
current_user = request.headers.get('Authorization')
if current_user in users:
if current_user == username or users[current_user]['role'] == 'admin':
return jsonify(users[username]), 200
return jsonify({'error': 'Access Denied'}), 403
return jsonify({'error': 'User not found'}), 404
@app.route('/login', methods=['POST'])
def login():
data = request.get_json()
username, password = data.get('username'), data.get('password')
if username in users and password == 'password123':
return jsonify({'token': 'your_access_token_here'}), 200
= return jsonify({'error': 'Invalid credentials'}), 401
if __name__ == '__main__':
app.run(debug=True)
In this example:
- Users can only access their own data
- Admins can access everything
- Unauthorised access is blocked
It’s simple... but it does the job.
Security Is a journey, not a Destination
That workshop didn’t just teach me about broken access control... it fundamentally changed how I think about writing code. Security isn’t a tick-box or a final step. It’s a mindset. A process. And it needs to be embedded right from the start.
If you’re building software, make security part of your foundation. It’s far easier to build secure systems from the ground up than to patch holes later.
If you want to dig deeper, the OWASP Top 10 is a brilliant resource for understanding today’s most common security risks.
Because if you’re not thinking about how to secure your app... someone else is already figuring out how to break it.